Sunday, December 11, 2011
Java Windows Azure Introduction
Tuesday, November 1, 2011
Install JDK 7 on Ubuntu Linux
Download JDK7: http://www.oracle.com/technetwork/java/javase/downloads/index.html.
Follow the steps to Installation the JDK on Linux Platforms, http://download.oracle.com/javase/7/docs/webnotes/install/linux/linux-jdk.html.
1. Download the file. Before the file can be downloaded, you must accept the license agreement. The archive binary can be installed by anyone (not only root users), in any location that you can write to. However, only the root user can install the JDK into the system location.
2. Change directory to the location where you would like the JDK to be installed. Move the .tar.gz archive binary to the current directory.
3. Unpack the tarball and install the JDK.
$ tar zxvf jdk-7u<version>-linux-i586.tar.gz
The Java Development Kit files are installed in a directory called jdk1.7.0_<version> in the current directory.
4. Delete the .tar.gz file if you want to save disk space.
Finally, config your javac on Ubuntu Linux
In Terminal, type the command to install the java alternative.
$sudo update-alternatives --install /usr/bin/javac javac /usr/lib/jvm/jdk1.7.0/bin/javac 1
Where:
/usr/lib/jvm/ is the directory the JDK installed, on step 2; such that javac should be at /usr/lib/jvm/jdk1.7.0/bin/javac.
The last number, 1, is an integer of "priority"; it should be work on any integer number.
If you have more than one javac installed, type te comand to select the active javac.
$sudo update-alternatives --config javac
Sunday, October 23, 2011
Java SE 7 Update 1 released
Details of the release notes, please refer: http://www.oracle.com/technetwork/java/javase/jdk7-relnotes-429209.html
Monday, October 17, 2011
Sort array of object using custom Comparator

import java.util.Arrays;
import java.util.Comparator;
public class SortComparator {
final static int NUMBER_OF_OBJ = 5;
public class MyClass {
String name;
int number;
MyClass(String n, int i){
name = n;
number = i;
}
}
public static void main(String[] args){
System.out.println("Sort Comparator");
SortComparator sortComparator = new SortComparator();
MyClass[] myClassArray = new MyClass[NUMBER_OF_OBJ];
myClassArray[0] = sortComparator.new MyClass("ABC", 9);
myClassArray[1] = sortComparator.new MyClass("Eric", 6);
myClassArray[2] = sortComparator.new MyClass("Java", 7);
myClassArray[3] = sortComparator.new MyClass("Android", 199);
myClassArray[4] = sortComparator.new MyClass("test", -3);
showMyClass(myClassArray);
Arrays.sort(myClassArray, comparatorName);
System.out.println("\nmyClassArray sorted by name");
showMyClass(myClassArray);
Arrays.sort(myClassArray, comparatorNumber);
System.out.println("\nmyClassArray sorted by number");
showMyClass(myClassArray);
}
static Comparator<? super MyClass> comparatorName
= new Comparator<MyClass>(){
public int compare(MyClass obj1, MyClass obj2) {
return String.valueOf(obj1.name).compareTo(obj2.name);
}
};
static Comparator<? super MyClass> comparatorNumber
= new Comparator<MyClass>(){
public int compare(MyClass obj1, MyClass obj2) {
return Integer.valueOf(obj1.number).compareTo(obj2.number);
}
};
static void showMyClass(MyClass[] mc){
for(int i = 0; i < mc.length; i++){
System.out.println(mc[i].name
+ " : "
+ mc[i].number);
}
}
}
Thursday, October 13, 2011
Sort Array
Example:

import java.lang.String;
import java.util.Arrays;
public class exSortArray{
public static void main(String[] args){
String src[] ={
"MNO",
"A",
"DEF",
"GHI",
"JKL",
"PQR",
"BC",
"STU",
"YZ",
"VWX"};
System.out.println("Sort Array Exerecise");
System.out.println();
System.out.println("Array before Sort");
showAll(src);
System.out.println();
Arrays.sort(src);
System.out.println("Array after Sort");
showAll(src);
}
static void showAll(String[] a){
for(int i = 0; i < a.length; i++){
System.out.println(i + ": " + a[i]);
}
}
}
Related Article:
- Sort array of object using custom Comparator
Tuesday, October 11, 2011
Copy Array

import java.lang.String;
public class exCopyArray{
public static void main(String[] args){
String src[] ={
"ABC",
"DEF",
"GHI",
"JKL",
"MNO",
"PQR",
"STU",
"VWX",
"YZ"};
System.out.println("Copy Array Exerecise");
showAll(src);
System.out.println();
String[] dest = new String[5];
System.arraycopy(src, //Copy from
2, //Source position
dest, //Copy to
0, //Destination position
5); //length to copy
showAll(dest);
}
static void showAll(String[] a){
System.out.println(a.toString());
for(int i = 0; i < a.length; i++){
System.out.println(i + ": " + a[i]);
}
}
}
Saturday, October 8, 2011
NetBeans IDE 7.1 Beta Released with JavaFX 2.0 supported
NetBeans IDE 7.1 Beta is available in English, Brazilian Portuguese, Japanese, Russian, and Simplified Chinese.
link: http://netbeans.org/community/releases/71/
Monday, October 3, 2011
Exercise on LinkedList
import java.util.LinkedList;
public class exLinkedList{
static LinkedList<String> myLinkedList;
public static void main(String[] args){
System.out.println("LinkedList Exerecise");
myLinkedList = new LinkedList<String>();
myLinkedList.add("ABCDEF");
myLinkedList.add("G");
myLinkedList.add("HIJ");
myLinkedList.add("KLM");
myLinkedList.add("NOPQRST");
myLinkedList.add("UVW");
myLinkedList.add("XYZ");
showAll();
System.out.println();
myLinkedList.removeFirst();
myLinkedList.removeLast();
myLinkedList.remove("KLM");
myLinkedList.remove("JAVA"); //Can't found!
showAll();
}
static void showAll(){
for(int i = 0; i < myLinkedList.size(); i++){
System.out.println(myLinkedList.get(i));
}
}
}

Saturday, October 1, 2011
Java The Complete Reference, 8th Edition
The Definitive Java Programming Guide
In Java: The Complete Reference, Eighth Edition, bestselling programming author Herb Schildt shows you everything you need to develop, compile, debug, and run Java programs. Updated for Java Platform, Standard Edition 7 (Java SE 7), this comprehensive volume covers the entire Java language, including its syntax, keywords, and fundamental programming principles. You'll also find information on key elements of the Java API library. JavaBeans, servlets, applets, and Swing are examined and real-world examples demonstrate Java in action. In addition, new Java SE 7 features such as try-with-resources, strings in switch, type inference with the diamond operator, NIO.2, and the Fork/Join Framework are discussed in detail.
Coverage includes:
- Data types and operators
- Control statements
- Classes and objects
- Constructors and methods
- Method overloading and overriding
- Interfaces and packages
- Inheritance
- Exception handling
- Generics
- Autoboxing
- Enumerations
- Annotations
- The try-with-resources statement
- Varargs
- Multithreading
- The I/O classes
- Networking
- The Collections Framework
- Applets and servlets
- JavaBeans
- AWT and Swing
- The Concurrent API
- Much, much more
About the Author
Thursday, September 29, 2011
Pre-Built Developer VMs, for Oracle VM VirtualBox.

Oracle have packaged pre-built Oracle VM VirtualBox appliances that you can download, install, and experience as a single unit. Just assemble the downloaded files (if needed), import into VirtualBox (available for free), import, and go!
Developer VMs are currently available in these flavors, with more to come.
- Java Development VM
- Database App Development VM
- SOA & BPM Development VM
- Enterprise Java Development VM
- Oracle WebCenter Portal Framework 11g Hands-on VM
- Oracle Solaris 11 Express Developer VM
- Oracle Solaris 11 Express Network Virtualization VM
- Oracle Tuxedo Web Application Server Demo VM
- Enterprise PHP Development VM
- Oracle Business Intelligence Enterprise Edition Plus VM
Know more>>
JavaOne 2011 app on mobile

The JavaOne application provides a comprehensive view of all things JavaOne— the flagship annual conference for Java developers worldwide ! taking place in San Francisco from October 2-6, 2011. Find out what's happening and where at JavaOne ‑all of the activities you need to attend, all of the people you want to meet—all on your mobile device.
available:
link: JavaOne Web Site
Wednesday, September 28, 2011
Implement Insertion Sort in Java
public class InsertionSort {
public static void main(String[] args) {
System.out.println("Hello, Java-Buddy!");
MyData myData = new MyData();
myData.show(); //Before sort
myData.InsertionSort();
myData.show(); //After sort
}
static class MyData {
final static int LENGTH = 10;
static int[] data = new int[LENGTH];
MyData(){
//Generate the random data
for (int i = 0; i < 10; i++) {
data[i] = (int)(100.0*Math.random());
}
}
void InsertionSort(){
int cur, j;
for (int i = 1; i < LENGTH; i++) {
cur = data[i];
j = i - 1;
while ((j >= 0) && (data[j] > cur)) {
data[j + 1] = data[j];
j--;
}
data[j + 1] = cur;
}
}
void show(){
for (int i = 0; i < 10; i++) {
System.out.print(data[i] + " ");
}
System.out.println("\n");
}
}
}

Sunday, September 25, 2011
Saturday, September 24, 2011
NetBeans: Setting Events With the Connection Wizard
Work on the exercise in last post "HelloNetBeans: Create Java Desktop Application using NetBeans IDE".
- Add a Button on Design Pane
- Right click on Button, Edit Text and enter "Click Me" as the text on the button.
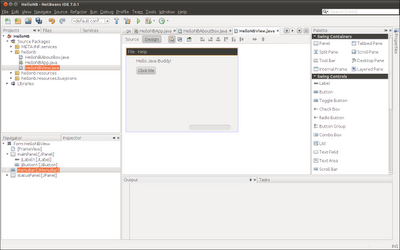
- Switch Connection Mode.

- Select the component (JButton1) that will fire the event, the selected component is highlighted in red when selected.
- Select the component (JLabel1) whose state you want to affect with the event.
- NetBeans will open Connection Wizard.
- Accept jButton1 as Source Component.
- Expand and select mouse -> mouseClicked as the source Events, with jButton1MouseClicked as Method Name in Event Handler Method.
- Click Next.

- Accept jLabel1 as Target Component.
- Select Set Property.
- Select text. and click Next.

- Select and Enter Value of any text you want, and click Finish.

- NetBeans will generate the code for you.

- Save and Run it again.

Friday, September 23, 2011
HelloNetBeans: Create Java Desktop Application using NetBeans IDE
- Select Categories of Java, and Projects of Java Desktop Application, and click Next.
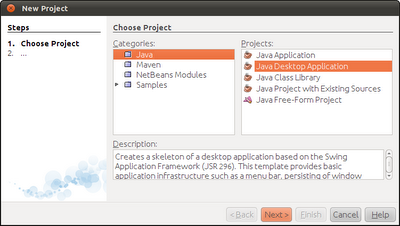
- Review Disclaimer and click Next.
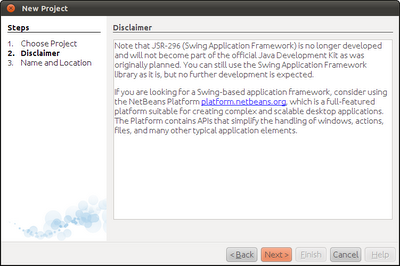
- Enter Project Name (HelloNB), and select Basic Application in Choose Application Shell box, and click Finish.
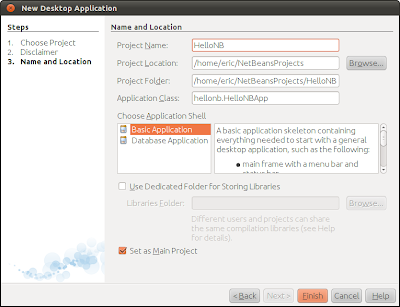
- Place a Label:
Like other GUI IDE, simple drag a Label (under Swing Controls) from the Palette pane over the Design Pane.
- Change the Label text:
Right click on the Label and select Edit Text.
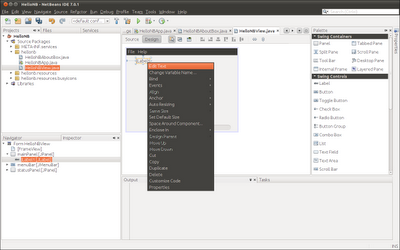
Enter the text.
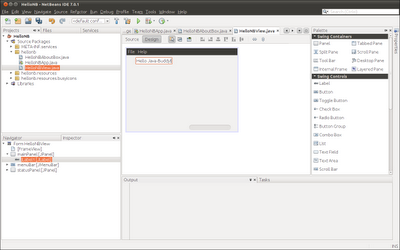
- Save All and Click the Green arrow icon to run the application.
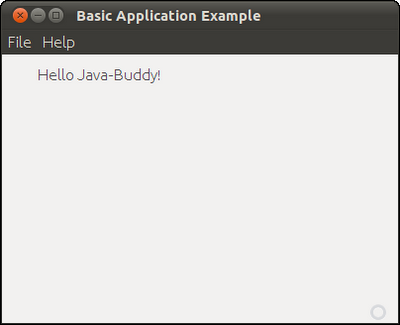
next:
- NetBeans: Setting Events With the Connection Wizard
Notice:
- If you cannot find Java Desktop Application in your NetBeans IDE, you can "Manually create GUI application of Hello World on NetBeans 7.2.1".
Wednesday, September 21, 2011
MyFirstApplet, run applet in HTML page
import javax.swing.JApplet;
import java.awt.Graphics;
public class MyFirstApplet extends JApplet {
public void paint(Graphics g) {
g.drawString("Hello, Java-Buddy!", 50, 50);
}
}
Compile it in command prompt:
javac MyFirstApplet.java

Create a HTML file MyFirstApplet.html, embed MyFirstApplet.class inside.
<html>
<head>
<title> Java-Buddy </title>
</head>
<body>
<hr>
<applet code = "MyFirstApplet.class" width = 300 height = 300 >
</applet>
<hr/>
</body>
</html>
Open MyFirstApplet.html in browser.

Tuesday, September 20, 2011
HelloJavaBuddy.java, first java code
Monday, September 19, 2011
Google APIs Client Library for Java announced, support OAuth 2.0
source: The official Google Code blog - Google APIs Client Library for Java: now with OAuth 2.0
Best Practices for Accessing Google APIs on Android
Ivor Horton's Beginning Java, Java 7 Edition
Ivor Horton's approach is teaching Java is so effective and popular that he is one of the leading authors of introductory programming tutorials, with over 160,000 copies of his Java books sold. In this latest edition, whether you're a beginner or an experienced programmer switching to Java, you'll learn how to build real-world Java applications using Java SE 7. The author thoroughly covers the basics as well as new features such as extensions and classes; extended coverage of the Swing Application Framework; and he does it all in his unique, highly accessible style that beginners love.
- Provides a thorough introduction to the latest version of the Java programming language, Java SE 7
- Introduces you to a host of new features for both novices and experienced programmers
- Covers the basics as well as new language extensions and classes and class methods
- Guides you through the Swing Application Framework for creating Swing apps
- Uses numerous step-by-step programming examples to guide you through the development process
There's no better way to get thoroughly up to speed on the latest version of Java than with Ivor Horton's latest, comprehensive guide.
NetBeans IDE 7.0.1 with JDK 7

The NetBeans IDE is an award-winning integrated development environment available for Windows, Mac, Linux, and Solaris. The NetBeans project consists of an open-source IDE and an application platform that enable developers to rapidly create web, enterprise, desktop, and mobile applications using the Java platform, as well as PHP, JavaScript and Ajax, Groovy and Grails, and C/C++.
NetBeans IDE 7.0 introduces language support for development to the Java SE 7 specification with JDK 7 language features. The release also provides enhanced integration with the Oracle WebLogic server, as well as support for Oracle Database and GlassFish 3.1. Additional highlights include Maven 3 and HTML5 editing support; a new GridBagLayout designer for improved Swing GUI development; enhancements to the Java editor, and more.
The latest available download is NetBeans IDE 7.0.1, which is an update to NetBeans IDE 7.0.
link: http://netbeans.org/community/releases/70/
how to:
- Create Java Desktop Application using NetBeans IDE
- Setting Events With the Connection Wizard
Sunday, September 18, 2011
Java Magazine for FREE
- Profiles of innovative Java applications
- Java technical how-to’s: Enterprise Java, New to Java, Rich Client, Polyglot Programming, and more
- Java community news: Java User Groups, JCP standards, and more
- Information about new Java books and conferences and events
http://www.oracle.com/technetwork/java/javamagazine/index.html
Java@Wiki

Java is a programming language originally developed by James Gosling at Sun Microsystems (which is now a subsidiary of Oracle Corporation) and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities. Java applications are typically compiled to bytecode (class file) that can run on any Java Virtual Machine (JVM) regardless of computer architecture. Java is a general-purpose, concurrent, class-based, object-oriented language that is specifically designed to have as few implementation dependencies as possible. It is intended to let application developers "write once, run anywhere." Java is currently one of the most popular programming languages in use, particularly for client-server web applications.
The original and reference implementation Java compilers, virtual machines, and class libraries were developed by Sun from 1995. As of May 2007, in compliance with the specifications of the Java Community Process, Sun relicensed most of its Java technologies under the GNU General Public License. Others have also developed alternative implementations of these Sun technologies, such as the GNU Compiler for Java, GNU Classpath, and Dalvik.
Ref: Wikipedia - Java (programming language)