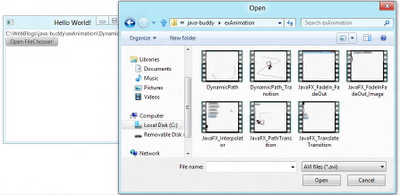
/* * To change this template, choose Tools | Templates * and open the template in the editor. */ package javafx_filechooser; import java.io.File; import javafx.application.Application; import javafx.event.ActionEvent; import javafx.event.EventHandler; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.control.Label; import javafx.scene.layout.StackPane; import javafx.scene.layout.VBox; import javafx.stage.FileChooser; import javafx.stage.Stage; /** * * @author Seven */ public class JavaFX_FileChooser extends Application { File file; /** * @param args the command line arguments */ public static void main(String[] args) { launch(args); } @Override public void start(Stage primaryStage) { primaryStage.setTitle("Hello World!"); final Label labelFile = new Label(); Button btn = new Button(); btn.setText("Open FileChooser'"); btn.setOnAction(new EventHandler<ActionEvent>() { @Override public void handle(ActionEvent event) { FileChooser fileChooser = new FileChooser(); //Set extension filter FileChooser.ExtensionFilter extFilter = new FileChooser.ExtensionFilter("AVI files (*.avi)", "*.avi"); fileChooser.getExtensionFilters().add(extFilter); //Show open file dialog file = fileChooser.showOpenDialog(null); labelFile.setText(file.getPath()); } }); VBox vBox = new VBox(); vBox.getChildren().addAll(labelFile, btn); StackPane root = new StackPane(); root.getChildren().add(vBox); primaryStage.setScene(new Scene(root, 300, 250)); primaryStage.show(); } }
Next:
- JavaFX 2.0: FileChooser, set initial directory from last directory
Related:
- Save file with JavaFX FileChooser
- Read text file with JavaFX FileChooser
- Use JavaFX FileChooser to open image file, and display on ImageView
- Simple example of DirectoryChooser
- Example of using Swing JFileChooser
thanks so much!!
ReplyDeleteworks perfect!
thank U very match! good job!)
ReplyDeleteok, but code
ReplyDeletefileChooser.showOpenDialog(null);
I think is bad solution, because you can open multiplies FileChooser dialogs - opening in no modal style.
And my question, if can I get Window from anywhere? Maybe from event?
Window is superclass of Stage, so you can use youre stage instead of null.
ReplyDeleteIt works. =)
thanks you very mat
ReplyDeleteplease can you make a simple mediaplayer that select a file file from the system and plays it
ReplyDelete